Currently in development.
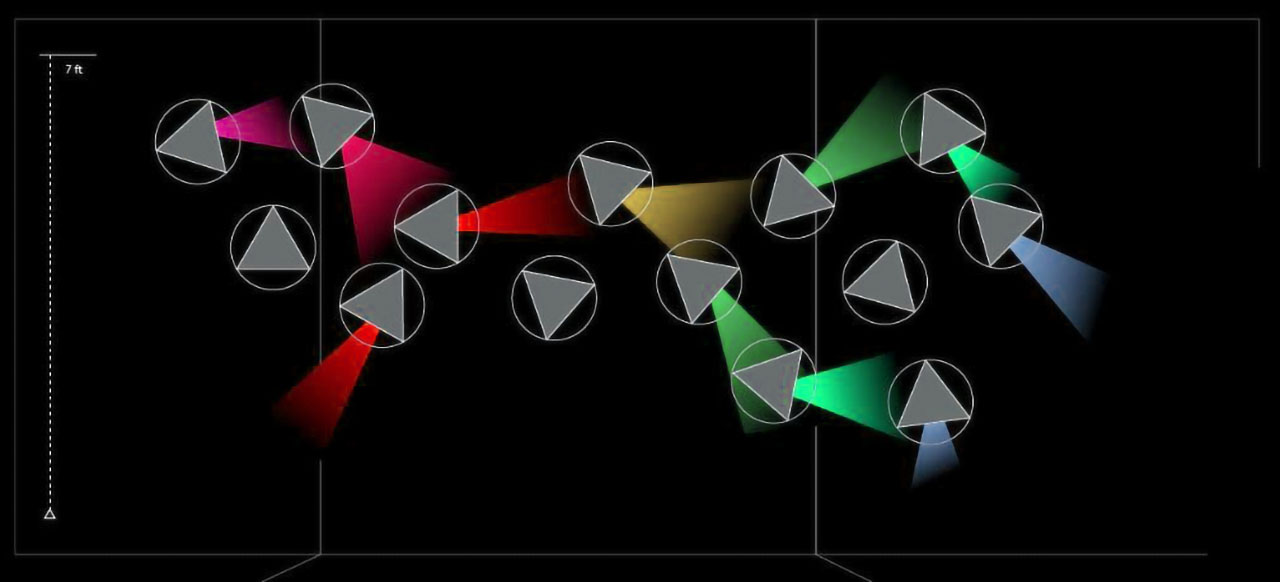
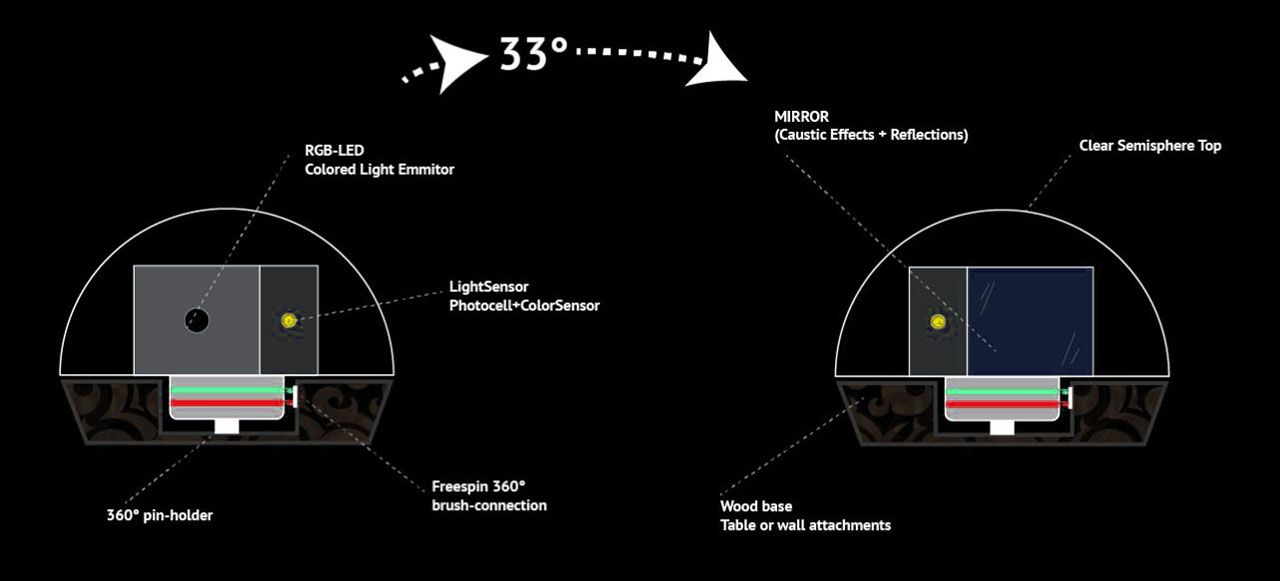
Coupled with an arduino or atTiny and capacitive sensing,
each module can trigger a rainbow effect by “letting go”
of the object after rotating it in place.
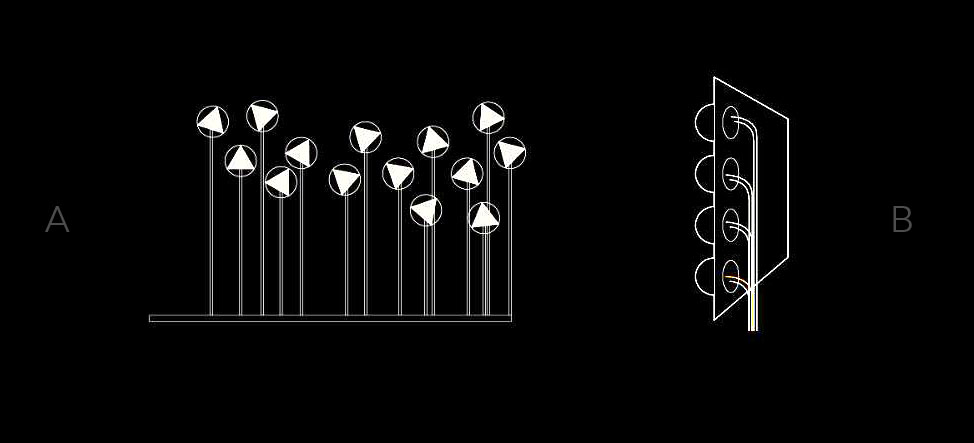
When module’s senses light -the color of light is also recognized
and the next in sequence is emmitted thereby continuing the relay of color.
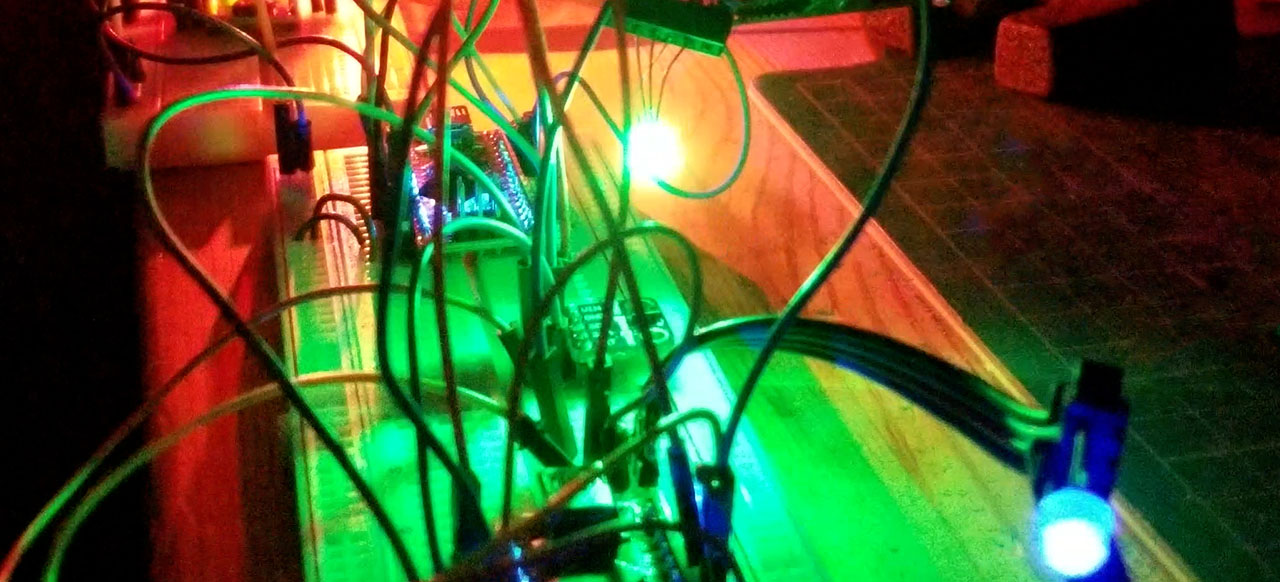
This Full-color LED RELAY is made possible with the APDS 9960 gesture sensor.
The code is designed to control an RGB LED light system, which changes its
colors based on ambient light levels and touch input. Here’s a detailed
breakdown of what it does:
Setup
- Libraries Included:
CapacitiveSensor
for touch sensing.Wire
andSparkFun_APDS9960
for handling the APDS-9960 sensor
- Global Variables:
cs_4_8
: Initializes the capacitive sensor with pins 4 and 8.apds
: Object for the APDS-9960 light sensor.ambient_light
,red_light
,green_light
,blue_light
: Variables to
store light sensor readings.touchTimeMultiplier
,touchThreshold
,ambLightThreshold
,ambLightCeiling
: Thresholds and multipliers for timing and sensing.rPin
,gPin
,bPin
: Pin assignments for the RGB LED.
- Setup Function:
- Initializes the RGB LED pins.
- Sets up serial communication if
debugSwitch
istrue
. - Initializes the APDS-9960 sensor and its light sensor.
- Calibrates the capacitive sensor.
Main Loop
- Reading Light Levels:
- Reads ambient, red, green, and blue light levels from the
APDS-9960 sensor.
- Reads ambient, red, green, and blue light levels from the
- Light Sensing Logic:
- Checks if ambient light is above a certain threshold (
ambLightThreshold
).
If so, it maps the light values to create a fading effect (fadeValue
) for the RGB LED. - Depending on the predominant color detected (red, green, blue), it
transitions to the next color in the ROYGBV (red, orange, yellow, green,
blue, violet) sequence.
- Checks if ambient light is above a certain threshold (
- Touch Sensing Logic:
- Detects touch using the capacitive sensor.
- If a touch is detected, it starts a timer (
startTime
) and calculates afadeTime
based on the duration of the touch andtouchTimeMultiplier
. - Randomly selects a color (red, orange, yellow, green, blue, violet) to fade to,
based onrandomSelect
.
- Fading Effect:
- Applies a fading effect to the RGB LED based on the selected color and the
fadeTime
.
- Applies a fading effect to the RGB LED based on the selected color and the
- Reset Logic:
- Resets the system if no touch is detected and the light sensing is off,
setting the RGB LED to white.
- Resets the system if no touch is detected and the light sensing is off,
- Debug Output:
- If
debugSwitch
is enabled, it prints sensor readings and states to the serial
monitor for debugging purposes.
- If
Key Points
- The capacitive sensor detects touch input to trigger color changes.
- The APDS-9960 sensor reads ambient light and adjusts the LED colors based
on light levels and specific color dominance. - The RGB LED transitions through colors in a sequence (ROYGBV) with fading effects.
- The system can be debugged via serial output if
debugSwitch
is enabled.
Overall, the code creates an interactive LED lighting system that responds to both ambient
light and touch, providing a dynamic and colorful display